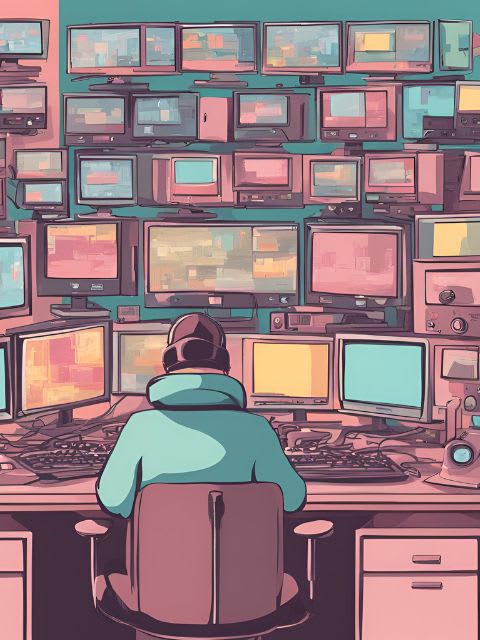
ESP32-CAM streaming
Getting the realtime video stream out of your ESP32-CAM should take little to no effort. Yet you won't find a single sketch in the entire internet that is less than a hundred lines of spaghetti code for camera pin assignment and web server configuration.
Beside this page of the internet, of course.
EspKit library
The EspKit library for Arduino defines a set of abstractions that make the ESP32 features easily accessible with few lines of code. Regarding the camera access, here's a list of what's included, to give you an idea:
- pin assignment
- sensor configuration
- fast jpeg decoding
- motion detection
- face detection & recognition
- object detection using Edge Impulse
Installation
Install the latest version of espkit
from the Arduino Library Manager.
MJPEG Stream
If you did not read the ESP32-CAM Quickstart tutorial already, it is a good time to do so, since it explains in detail how to configure and initialize the camera instance. After that, you can connect to WiFi and run an HTTP server on the ESP32-CAM to serve the stream.
/**
* See camera stream in MJPEG format.
* Useful to capture images, too.
*
* Set Tools > Core Debug Level > Info
*/
#include <espkit.h>
#include <espkit/wifikit.h>
#include <espkit/mdnskit.h>
#include <espkit/camera-mjpeg.h>
void setup() {
Serial.begin(115200);
ESP_LOGI("App", "Mjpeg Stream example");
// try to connect to WiFi
// default timeout is 8 seconds
// default retries is 2
wifikit.to("SSID", "PASSWORD").connect();
wifikit.raise();
ESP_LOGI("APP", "Connected to WiFi with IP %s", wifikit.ip.c_str());
// set hostname
// camera will be accessible at http://esp32cam.local
mdnskit.begin("esp32cam");
// configure the camera
camera.config.pinout.wroom_s3();
camera.config.pixformat.jpeg();
camera.config.quality.high();
camera.config.resolution.square();
// init camera and raise error if something goes wrong
camera.begin();
camera.raise();
// init server
mjpegServer.listenOn(80);
mjpegServer.begin();
ESP_LOGI("APP", "Server is running...");
}
void loop() {
// MJPEG HTTP server runs in a background thread
}
/**
* See camera stream in MJPEG format.
* Useful to capture images, too.
*
* Set Tools > Core Debug Level > Info
*/
#include <espkit.h>
#include <espkit/wifikit.h>
#include <espkit/mdnskit.h>
#include <espkit/camera-mjpeg.h>
void setup() {
Serial.begin(115200);
ESP_LOGI("App", "Mjpeg Stream example");
// try to connect to WiFi
// default timeout is 8 seconds
// default retries is 2
wifikit.to("SSID", "PASSWORD").connect();
wifikit.raise();
ESP_LOGI("APP", "Connected to WiFi with IP %s", wifikit.ip.c_str());
// set hostname
// camera will be accessible at http://esp32cam.local
mdnskit.begin("esp32cam");
// configure the camera
camera.config.pinout.wroom_s3();
camera.config.pixformat.jpeg();
camera.config.quality.high();
camera.config.resolution.square();
// init camera and raise error if something goes wrong
camera.begin();
camera.raise();
// init server
mjpegServer.listenOn(80);
mjpegServer.begin();
ESP_LOGI("APP", "Server is running...");
}
void loop() {
// MJPEG HTTP server runs in a background thread
}
The server runs in a separate thread, so in the loop()
you can still execute your custom code.
If you open the Serial Monitor, you will read some messages, like the following screen:

[HTTP] MJPEG server address: http://192.168.1.155:80

The IP address will vary depending on your Wi-Fi connection. If your router supports mDNS, you will also be able to access the stream at http://esp32cam.local
The server serves two endpoints:
/
to display a full HTML web page (to also capture frames, see below)/raw
will serve the raw MJPEG stream (to open e.g. in VLC)
Click the button (or press Shift + s
) to save a still picture. This is useful to collect images to train an object detection model.