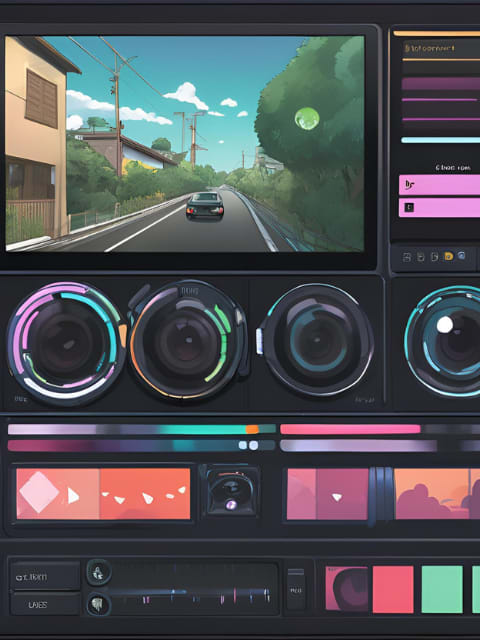
ESP32-CAM webserver
Good chances are the first sketch you loaded on your ESP32-CAM is the CameraWebServer
sketch, a gigantic example of spaghetti code that shouldn't have been ever written. This tutorial gives a total restyling to that sketch, both in terms of code and UI.
EspKit library
The EspKit library for Arduino defines a set of abstractions that make the ESP32 features easily accessible with few lines of code. Regarding the camera access, here's a list of what's included, to give you an idea:
- pin assignment
- sensor configuration
- fast jpeg decoding
- motion detection
- face detection & recognition
- object detection using Edge Impulse
Installation
Install the latest version of espkit
from the Arduino Library Manager.
To configure the ESP32-CAM sensor to match your specific setup, you can load the CameraWebServer sketch. This is similar to the MJPEG streaming sketch we saw in a previous tutorial, but adds a list of controls you can use to tweak the sensor settings.
Here's the code.
/**
* CameraWebServer example with a new style
*
* Set Tools > Core Debug Level > Info
*/
#include <espkit.h>
#include <espkit/wifikit.h>
#include <espkit/mdnskit.h>
#include <espkit/camera-webserver.h>
void setup() {
Serial.begin(115200);
ESP_LOGI("App", "CameraWebServer example");
// try to connect to WiFi
// default timeout is 8 seconds
// default retries is 2
wifikit.to("SSID", "PASSWORD").connect();
wifikit.raise();
ESP_LOGI("APP", "Connected to WiFi with IP %s", wifikit.ip.c_str());
// set hostname
// camera will be accessible at http://esp32cam.local
mdnskit.begin("esp32cam");
// configure the camera
camera.config.pinout.prompt();
camera.config.pixformat.jpeg();
camera.config.quality.high();
camera.config.resolution.vga();
// init camera and raise error if something goes wrong
camera.begin();
camera.raise();
// init server
cameraWebServer.listenOn(80);
cameraWebServer.begin();
}
void loop() {
// Camera HTTP server runs in a background thread
}
/**
* CameraWebServer example with a new style
*
* Set Tools > Core Debug Level > Info
*/
#include <espkit.h>
#include <espkit/wifikit.h>
#include <espkit/mdnskit.h>
#include <espkit/camera-webserver.h>
void setup() {
Serial.begin(115200);
ESP_LOGI("App", "CameraWebServer example");
// try to connect to WiFi
// default timeout is 8 seconds
// default retries is 2
wifikit.to("SSID", "PASSWORD").connect();
wifikit.raise();
ESP_LOGI("APP", "Connected to WiFi with IP %s", wifikit.ip.c_str());
// set hostname
// camera will be accessible at http://esp32cam.local
mdnskit.begin("esp32cam");
// configure the camera
camera.config.pinout.prompt();
camera.config.pixformat.jpeg();
camera.config.quality.high();
camera.config.resolution.vga();
// init camera and raise error if something goes wrong
camera.begin();
camera.raise();
// init server
cameraWebServer.listenOn(80);
cameraWebServer.begin();
}
void loop() {
// Camera HTTP server runs in a background thread
}